- Prerequisites
- 1. Send a GET Request
- 2. Identify the HTML Elements to Scrape
- 3. Getting HTML Element Attributes
- 4. Controlling Timeouts
- 5. Extract Data
- 6. Handling Dynamic Content
- 7. Handling Pagination
- 8. Data Cleaning and Processing
- 9. Saving Data to a Data Frame
- 10. Exporting Data Frame to a CSV File
- 11. Error Handling and Debugging
- How Can I Avoid Being Blocked While Scraping a Website?
- How to Use Proxies With rvest During Scraping
- Rotating Proxies to Avoid Detection
- Frequently Asked Questions
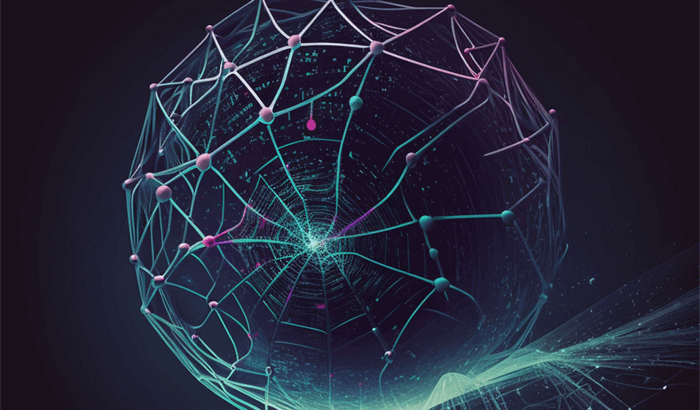
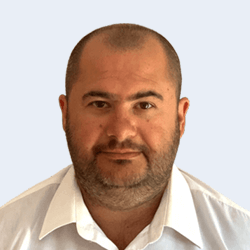
Web scraping has become an essential tool for data enthusiasts, researchers, and developers to gather information from the web. With the vast amount of data available online, web scraping allows users to automate the collection process, making it easier to analyze, visualize, or store data for further use. In this article, we’ll explore how to perform web scraping using the R language and the powerful rvest
package. We'll walk you through various aspects of web scraping in R: From the basics of sending GET requests to handling pagination, dynamic content, and proxies, including how to use Infatica proxies to avoid getting blocked while web scraping.
Prerequisites
Before starting with web scraping, the first step is to set up your development environment by installing R and RStudio. R is a powerful programming language designed for statistical computing and data analysis, while RStudio provides a user-friendly interface to write and run R code efficiently.
Install R and RStudio
Install R: Visit the official R website and download the version compatible with your operating system (Windows, macOS, or Linux). Follow the installation instructions, and once completed, R should be ready to use.
Install RStudio: After installing R, download and install RStudio from the RStudio website. It’s a feature-rich IDE (Integrated Development Environment) designed specifically for R, offering powerful tools like code suggestions, built-in debugging, and support for package management..
Once both are installed, you can launch RStudio, which will automatically detect your R installation.
Install Required Packages
To start your R project, you'll need several packages. The primary one is rvest
, which simplifies web scraping tasks. Additionally, you may require other packages like xml2
for parsing HTML content, dplyr
for data manipulation, and stringr
for handling text.
You can install these packages by running the following commands in your RStudio console:
install.packages("rvest")
install.packages("xml2")
install.packages("dplyr")
install.packages("stringr")
These packages will be downloaded from CRAN and installed on your system. If they’re already installed, R will notify you and skip the installation.
Load Required Libraries
After installing the necessary packages, you'll need to load them into your R session before you can use their functions. This can be done with the library()
function. Here's how to load the required libraries:
library(rvest)
library(xml2)
library(dplyr)
library(stringr)
Loading these libraries ensures that all the functions provided by the packages are available for use in your script. Each library plays a crucial role in the web scraping operation: the rvest
library for extracting information, xml2
for parsing the structure of the HTML document, and dplyr
and stringr
for cleaning and structuring the scraped data.
Scraping data with R and rvest
1. Send a GET Request
To begin web scraping in R, you must first retrieve the webpage's content using a GET request. The rvest
package provides the read_html()
custom function, which sends a request to the given URL and retrieves the HTML page.
library(rvest)
# URL of the webpage to scrape
url <- "https://example.com"
# Send GET request and retrieve the webpage content
webpage <- read_html(url)
In this snippet, the following function – read_html()
– sends a request to the URL and stores the response (HTML documents) in the webpage
object. This content can now be parsed to extract data.
2. Identify the HTML Elements to Scrape
Once you have the HTML content, you need to identify which HTML element contains the data you want to extract. This can be done by inspecting the webpage with your browser's developer tools (right-click on the page and select "Inspect").
For instance, if the page contains page titles within <h2>
tags with a class of "article-title", you can target those elements with the following code:
# Extracting all <h2> tags with the class 'article-title'
titles <- webpage %>% html_elements("h2.article-title") %>% html_text()
# View the extracted titles
titles
Here, html_elements()
selects all <h2>
tags with the class article-title
, and html_text()
extracts the visible text inside those tags. The %>%
is a pipe operator that allows chaining commands for cleaner code.
3. Getting HTML element attributes
When web scraping, you may want to extract not only the text format but also the attributes (like links or image sources) of raw HTML elements. This can be done using either specific CSS selectors or XPath expressions.
CSS selectors are patterns used to select HTML elements based on their classes, IDs, or tags, while XPath is a syntax for navigating through elements and attributes in an XML document.
Here's a code snippet that uses a CSS Selector:
# Extracting URLs from 'a' (anchor) tags using CSS selectors
links <- webpage %>% html_elements("a") %>% html_attr("href")
# View the extracted links
links
And a code snippet that uses XPath:
# Extracting URLs using XPath expressions
links_xpath <- webpage %>% html_elements(xpath = "//a") %>% html_attr("href")
# View the extracted links
links_xpath
In both examples, html_attr("href")
extracts the href
attribute, which contains the URLs of the links.
4. Controlling Timeouts
Some websites may take longer to respond, so you might need to control timeouts for your GET requests. While rvest
itself doesn’t have built-in timeout controls, you can use the httr
package, which integrates well with rvest
to handle more complex requests.
library(httr)
# Set a custom timeout of 10 seconds using the httr package
response <- GET(url, timeout(10))
# Parse the response content with rvest
webpage <- content(response, as = "text") %>% read_html()
In this snippet, GET()
sends the request with a 10-second timeout limit, ensuring the request won’t hang indefinitely. The response is then parsed using read_html()
.
5. Extract Data
After identifying the elements and handling timeouts, you can extract various types of data such as text, links, and HTML tables. Let’s explore each:
Example A: Extract text: To extract visible text from an HTML element (like a paragraph or a header):
# Extract text from <p> tags
paragraphs <- webpage %>% html_elements("p") %>% html_text()
# View the extracted paragraphs
paragraphs
Here, the html_text()
function retrieves the text content from all <p>
tags.
Example B: Extract links: To extract hyperlinks (URLs) from anchor (<a>
) tags:
# Extract href attributes (links) from <a> tags
links <- webpage %>% html_elements("a") %>% html_attr("href")
# View the extracted links
links
In this example, html_attr("href")
extracts the URLs stored in the href
attribute of anchor tags.
Example C: Extract tables: A web page may often contain tabular data in <table>
tags. The html_table()
function automatically converts these tables into a data frame.
# Extract table data
tables <- webpage %>% html_elements("table") %>% html_table()
# View the first table
tables[[1]]
The html_table()
function returns a list of data frames, each representing a table on the webpage. You can access individual tables using list indexing ([[1]]
for the first table).
6. Handling Dynamic Content
Dynamic websites load data via JavaScript, making it difficult to scrape using traditional HTML methods. In these cases, you can use tools like Selenium or check if the site provides an API for data access.
To handle dynamic content in R, the RSelenium package can be used to simulate a browser and scrape the rendered content.
library(RSelenium)
# Start RSelenium server and browser
rD <- rsDriver(browser = "firefox", port = 4545L)
remDr <- rD$client
# Navigate to the webpage
remDr$navigate("https://example.com")
# Wait for dynamic content to load, then get the page source
Sys.sleep(5) # wait for JavaScript to load content
webpage <- remDr$getPageSource()[[1]] %>% read_html()
# Extract data as usual
data <- webpage %>% html_elements("div.dynamic-content") %>% html_text()
remDr$close()
This code uses RSelenium
to open a Firefox browser, load a webpage, and wait for dynamic content to be loaded before scraping the HTML file. You can then scrape the dynamic elements just like regular static ones.
7. Handling Pagination
Many websites display data across multiple pages, requiring you to scrape data from multiple URLs. Pagination can be handled by iterating through the pages and scraping each one individually.
library(rvest)
# Base URL of the website
base_url <- "https://example.com/page="
all_data <- list()
# Loop through multiple pages (e.g., 1 to 5)
for (i in 1:5) {
# Construct the full URL for each page
url <- paste0(base_url, i)
# Send GET request and scrape the data from each page
webpage <- read_html(url)
# Extract the desired data from the page
page_data <- webpage %>% html_elements(".data-item") %>% html_text()
# Append the scraped data to the list
all_data <- append(all_data, page_data)
}
# View all collected data
all_data
This loop constructs URLs for different pages by changing the page number in the URL query string. It collects data from each page and appends it to a list for processing later.
8. Data Cleaning and Processing
Once data is scraped, it often requires cleaning and formatting before further analysis. You can use packages like dplyr
and stringr
to clean and process the data.
library(dplyr)
library(stringr)
# Sample scraped data
scraped_data <- c(" Item 1 ", "\nItem 2", "Item 3 ")
# Clean the data by removing unnecessary whitespace and newline characters
clean_data <- scraped_data %>%
str_trim() %>% # Trim leading and trailing whitespace
str_remove_all("\n") %>% # Remove newline characters
str_to_title() # Convert text to title case
# View cleaned data
clean_data
This code cleans scraped data by trimming excess whitespace, removing newline characters, and converting the text to title case using functions from the stringr
package.
9. Saving data to a data frame
Once you have cleaned the data, you may want to store it in a data frame for easier manipulation and analysis. The data.frame()
function in R allows you to combine different variables into a structured format.
# Scraped data (e.g., names and prices of products)
names <- c("Product A", "Product B", "Product C")
prices <- c(10.99, 15.49, 7.99)
# Create a data frame
product_data <- data.frame(Name = names, Price = prices)
# View the data frame
product_data
This code creates a data frame where each column represents a different attribute (e.g., Name
and Price
), making it easier to work with the scraped data.
10. Exporting data frame to a CSV file
Once the data is stored in a data frame, you can easily export it to a CSV file for future use or sharing. The write.csv()
function in R allows you to export the data frame.
# Export the data frame to a CSV file
write.csv(product_data, "product_data.csv", row.names = FALSE)
This code exports the product_data
data frame to a file named product_data.csv
in the current working directory. The row.names = FALSE
argument ensures that row numbers aren’t included in the file.
11. Error Handling and Debugging
Data scraping is often prone to errors due to issues such as broken links, missing values, or slow response times. You can use tryCatch()
in R to handle these errors gracefully and ensure your script continues running.
# Example of error handling with tryCatch
scrape_page <- function(url) {
tryCatch({
# Attempt to scrape the webpage
webpage <- read_html(url)
data <- webpage %>% html_elements(".data-item") %>% html_text()
return(data)
}, error = function(e) {
# Print error message and return NULL in case of an error
message("Error scraping: ", url, "\n", e)
return(NULL)
})
}
# Test the function with a valid and an invalid URL
valid_data <- scrape_page("https://example.com")
invalid_data <- scrape_page("https://invalid-url.com")
In this example, tryCatch()
attempts to scrape a webpage and handles any errors by printing a message and returning NULL
instead of causing the script to fail. This makes your web scraper more robust and able to handle unexpected issues.
How Can I Avoid Being Blocked While Scraping a Website?
While you’re performing web scraping, especially at scale, websites often employ mechanisms to detect and block web scraping attempts. These include rate limiting, IP blocking, CAPTCHA challenges, or even user-agent detection. To mitigate these issues, rotating proxies like those offered by Infatica can be incredibly useful.
Infatica provides a pool of residential proxies that enable you to rotate IP addresses during web scraping in R, making it appear as though requests are coming from different real users around the world. This helps you avoid detection and blocks because:
IP Rotation: Constantly changing IP addresses prevent websites from recognizing your repeated access attempts from the same IP, which is a common red flag for automated web scraping.
Residential IPs: Unlike data center proxies, residential IPs are linked to real devices, making them harder for websites to detect and block.
Geolocation: Infatica proxies allow you to access location-restricted content by using IP addresses from specific countries or regions.
Bypassing CAPTCHAs: Many web pages display CAPTCHA challenges after a certain number of requests. By rotating proxies, you reduce the likelihood of being flagged and presented with CAPTCHAs.
How to Use Proxies With rvest During Scraping
You can integrate proxies into your scraping workflow by configuring the request to use them. R’s httr package provides a way to send web requests via a proxy, and this can be combined with rvest
.
To use proxies with rvest
, you can leverage the httr
package's use_proxy()
function, which sets the proxy server for the request.
library(rvest)
library(httr)
# Define the proxy (using Infatica proxy details)
proxy_ip <- "proxy_ip_address"
proxy_port <- 8080
proxy_username <- "your_username"
proxy_password <- "your_password"
# URL of the webpage to scrape
url <- "https://example.com"
# Use httr to send a GET request through a proxy
response <- GET(url, use_proxy(proxy_ip, proxy_port, proxy_username, proxy_password))
# Parse the HTML content from the response using rvest
webpage <- content(response, as = "text") %>% read_html()
# Extract the desired data (example: extracting text from <p> tags)
data <- webpage %>% html_elements("p") %>% html_text()
# View the extracted data
print(data)
Here’s what’s happening:
use_proxy()
: This function from the `httr` package allows you to specify the IP address and port of the proxy server. For proxies that require authentication, you also pass the username and password.GET()
: TheGET()
function sends a request through the specified proxy server to the URL you want to scrape.content()
: This retrieves the content of the webpage, which is then parsed by the rvest function usingread_html()
.
Rotating Proxies to Avoid Detection
Infatica provides a pool of proxies, which can be rotated after each request or at certain intervals. You can automate the process of switching proxies to further minimize the risk of being blocked.
library(rvest)
library(httr)
# List of proxies (from Infatica pool)
proxies <- list(
list(ip = "proxy1_ip", port = 8080, username = "user1", password = "pass1"),
list(ip = "proxy2_ip", port = 8080, username = "user2", password = "pass2"),
list(ip = "proxy3_ip", port = 8080, username = "user3", password = "pass3")
)
# URL of the webpage to scrape
url <- "https://example.com"
# Function to scrape data using rotating proxies
scrape_with_proxy <- function(url, proxy) {
response <- GET(url, use_proxy(proxy$ip, proxy$port, proxy$username, proxy$password))
webpage <- content(response, as = "text") %>% read_html()
data <- webpage %>% html_elements("p") %>% html_text()
return(data)
}
# Loop through proxies and scrape data
all_data <- list()
for (proxy in proxies) {
data <- scrape_with_proxy(url, proxy)
all_data <- append(all_data, data)
}
# View all scraped data
all_data
In this code, the proxies are rotated by iterating through a list of proxy servers, ensuring that requests are made using different IPs to avoid detection.
Frequently Asked Questions
The main steps of web scraping with R are:
- Identify the target web page and the data you want to extract.
- Inspect the HTML structure of the entire website and locate the elements that contain the data.
- Use rvest functions to read the HTML code, select elements, and extract the data.
- Format and clean the data as needed.
- Store the data in a data frame or export it to a file.