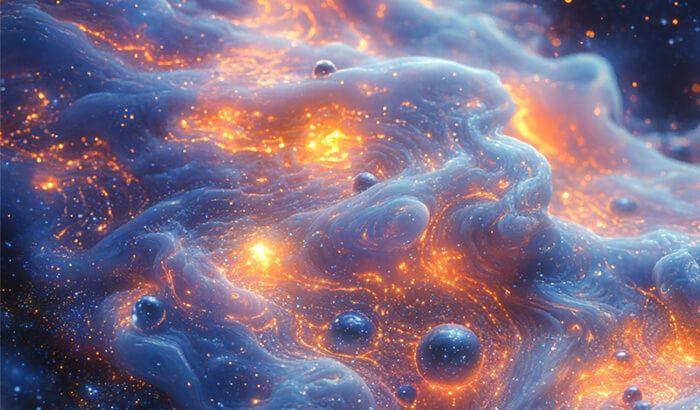
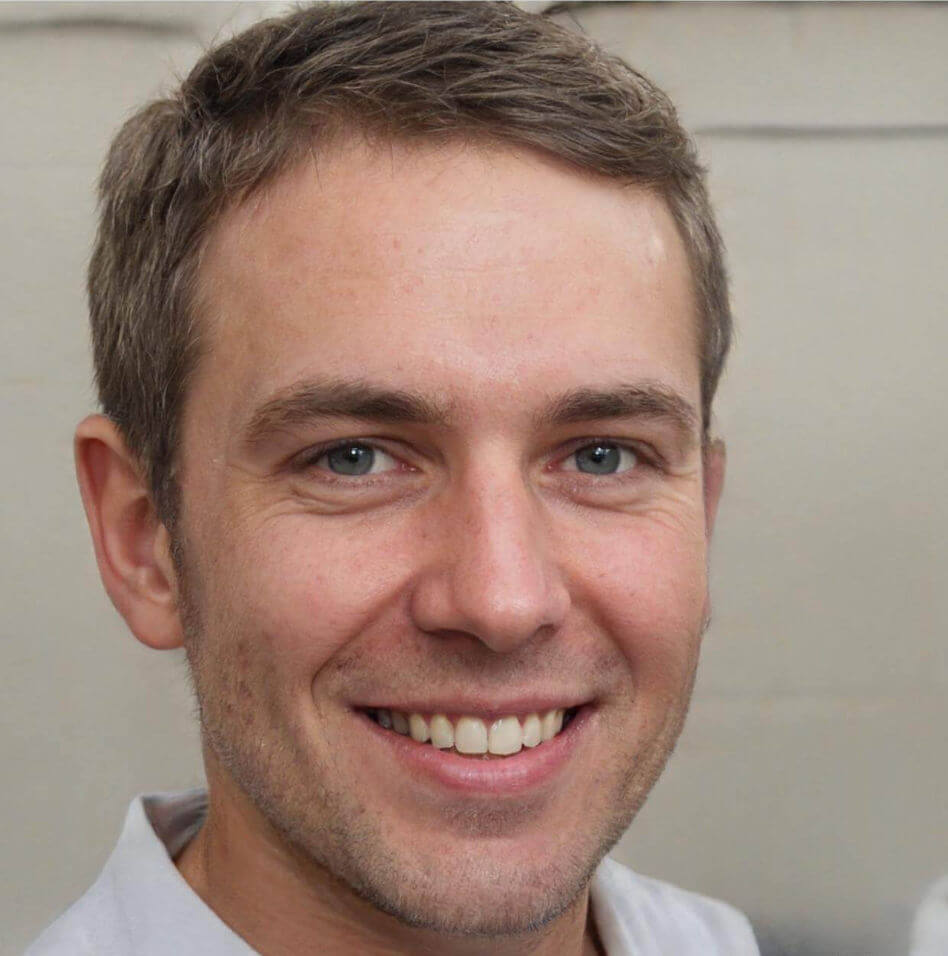
PHP is a widely-used scripting language that excels in web development, making it an excellent choice for all the data collection tasks like web scraping. In this article, you'll discover the fundamentals of web scraping with PHP, guiding you through everything from setting up your development environment to sending HTTP requests and extracting content from any dynamic website. We’ll cover essential libraries like cURL and Symfony Panther, providing practical examples for parsing HTML, handling pagination, and providing the final code for implementing rotating proxies to ensure successful data retrieval.
Is PHP Suitable for Web Scraping?
PHP is quite capable for scraping static websites. It has built-in functions like file_get_contents
and libraries such as cURL
that make it easy to fetch HTML content. The DOMDocument
class is useful for parsing HTML and extracting data. For simple pages that don’t rely heavily on JavaScript code for rendering content, PHP is often a practical choice, particularly when integrated into a backend system where PHP is already in use.
However, when dealing with more dynamic websites that rely on JavaScript for rendering, PHP can struggle. Unlike programming languages like JavaScript, which can run in the browser or be handled by tools like Puppeteer, or Python, which has libraries like Selenium and Playwright, PHP lacks native tools to interact directly with a page’s JavaScript. For these web scraping cases, developers may need to integrate PHP with a headless browser or rely on APIs, which can complicate the setup.
Libraries for PHP Web Scraping
Library | Description | Pros | Cons | Best for |
---|---|---|---|---|
cURL | A built-in PHP library for sending HTTP requests. Can retrieve HTML and data from websites. | Native to PHP. Flexible with HTTP methods. High control over requests | Limited HTML parsing. Requires handling cookies and sessions manually | Simple scraping tasks |
Goutte | Built on Guzzle and Symfony DomCrawler. Provides easy scraping with support for CSS selectors. | Intuitive API. CSS selector support. Handles redirects, cookies | Limited JavaScript support. Dependent on Guzzle and Symfony components | Static pages with complex HTML |
Symfony DomCrawler | Part of the Symfony components; allows parsing and navigating HTML documents using CSS selectors. | Easy HTML navigation. Integrates well with other Symfony components | No HTTP client (requires Guzzle or similar). Limited JavaScript support | Parsing and scraping simple HTML |
PHP Simple HTML DOM Parser | Parses HTML and provides an easy API similar to jQuery. | Simple syntax. Good CSS selector support. Lightweight | Slower with large HTML. Limited JavaScript and AJAX handling | Basic scraping with CSS selectors |
Panther | Uses headless Chrome to interact with and scrape JavaScript-heavy pages, part of Symfony. | Full JavaScript support. Headless browser automation - Screenshot support |
Heavy on resources. Requires installation of ChromeDriver | JavaScript-heavy and dynamic sites |
ReactPHP HTTP Client | Asynchronous HTTP client based on ReactPHP, suitable for handling multiple requests simultaneously. | Non-blocking, asynchronous requests. Fast for many concurrent requests | Limited HTML parsing capabilities. Higher learning curve for async patterns | High-frequency, concurrent requests |
Step-by-step by guide on Web Scraping with PHP
Step 1: Set Up Your PHP File
To start, create a new PHP file where your scraper’s code will live. This file will contain all the code needed to make HTTP requests, parse data, and store the results. You can name it something relevant, like scraper.php
. At the top of this file, start with the <?php
tag to begin writing PHP code, which is necessary for the web server to interpret your code snippet correctly. You might also want to set error reporting options to help with debugging while you work:
<?php
// Enable error reporting for debugging
error_reporting(E_ALL);
ini_set('display_errors', 1);
Step 2: Initialize cURL
To scrape a website with PHP, you’ll use the cURL library, which allows your code to make HTTP requests to any URL. Begin by initializing a new cURL session with curl_init()
. This function prepares the cURL library to start a request, returning a handle that you’ll use throughout the session. Assign this handle to a variable (e.g., $ch
) so you can configure and execute the request in the following steps.
// Initialize a new cURL session
$ch = curl_init();
Step 3: Set cURL Options
With the cURL session initialized, the next step is to set options that define how the request behaves. You’ll use curl_setopt()
to configure these options, including the URL you want to scrape and other key settings. Set the CURLOPT_URL
option with the target URL, and enable CURLOPT_RETURNTRANSFER
to store the response as a variable instead of printing it directly. You may also want to set CURLOPT_USERAGENT
to specify a user-agent string, making the request appear as if it’s coming from a browser.
// Set cURL options
curl_setopt($ch, CURLOPT_URL, "https://example.com");
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERAGENT, "Mozilla/5.0 (compatible; PHP Web Scraper)");
Step 4: Execute the cURL Session
After setting up your cURL options, you’re ready to execute the session and retrieve data from the target web page. Use curl_exec()
with your cURL handle to send the HTTP request. This function returns the HTML content of the current page as a string, which you can assign to a variable (e.g., $response
). This response will contain all the HTML code that you’ll parse and process in the upcoming steps.
// Execute the cURL session and store the response
$response = curl_exec($ch);
Step 5: Check for HTTP Errors
Once you’ve executed the cURL session, it’s a good practice to check for any HTTP errors. If the target URL fails to load or responds with an error (like a 404 or 500 status code), you’ll want to handle it gracefully rather than continuing with invalid data. Use curl_errno()
to check if any errors occurred during the request, and curl_getinfo()
to retrieve the HTTP status code. If an error is detected, you can display an error message and exit the script.
// Check for cURL errors
if (curl_errno($ch)) {
echo 'cURL error: ' . curl_error($ch);
} else {
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
if ($httpCode !== 200) {
echo "HTTP error: " . $httpCode;
}
}
Step 6: Parse HTML Content
Now that you have the HTML content stored in $response
, the next step is to parse it to locate specific elements. PHP’s DOMDocument
class is a useful tool for working with HTML, allowing you to load the HTML content and navigate the DOM tree. Start by creating a new `DOMDocument` object and loading the HTML content using loadHTML()
. Suppress errors with @
in case of malformed HTML, which is common in scraping data.
// Create a DOMDocument and load HTML content
$dom = new DOMDocument();
@$dom->loadHTML($response);
Step 7: Locate Specific Elements
With the HTML loaded into DOMDocument
, you can now navigate the DOM tree to locate specific elements. Use DOMXPath
, which enables you to query the document with XPath expressions, a powerful way to target elements by tag name, class, ID, or other attributes. Start by creating a DOMXPath
client object and passing in your DOMDocument
. You can then use the query()
method to select elements, such as all <div>
tags with a certain class.
// Create a DOMXPath instance and locate elements
$xpath = new DOMXPath($dom);
$elements = $xpath->query("//div[@class='example-class']");
Step 8: Extract Data
After locating the specific elements with DOMXPath
, you can proceed to extract the desired data from them. This step involves iterating over the DOMNodeList
returned by the query()
method and accessing the relevant properties or methods to retrieve the information you need. For example, you might want to get the text content of each element, such as the href
attribute for links. You can store this extracted data in an array for further processing.
// Extract data from located elements
$data = [];
foreach ($elements as $element) {
$data[] = $element->textContent; // Get the text content of each element
}
Step 9: Store Scraped Data
Once you have extracted the relevant data, the next step is to store it for later use. Depending on your requirements, you might choose to save the data in a variety of formats, such as a database, a CSV file, or even a simple text file. For instance, if you opt to save the data in a CSV file, you can use PHP's built-in fputcsv()
function, which writes an array as a line to a file. First, open a file for writing, then loop through your data array and write each piece of data to the file.
// Open a file for writing
$file = fopen("scraped_data.csv", "w");
// Write each data entry to the file
foreach ($data as $entry) {
fputcsv($file, [$entry]); // Write as a single column in CSV
}
// Close the file
fclose($file);
Step 10: Clean Up
After storing your scraped data, it's important to perform some clean-up tasks to ensure your script runs efficiently and doesn't leave any resources hanging. Begin by closing the cURL session using curl_close()
to free up the resources associated with it. This step is crucial for maintaining optimal performance, especially if you're running multiple web scrapers or making frequent requests. Additionally, you might want to unset any variables that are no longer needed to further free up memory.
// Close the cURL session
curl_close($ch);
// Optionally unset variables
unset($response, $data);
Step 11: Test Your Scraper
With your web scraper fully implemented, it’s time to test it to ensure it works as expected. Begin by running the script and observing the output. Check if the data is being scraped accurately from the target website and stored correctly in your chosen format. Pay attention to any error messages or warnings, and make sure to validate the contents of the scraped data. If the data isn’t coming through as anticipated, review the earlier steps to troubleshoot issues, such as incorrect XPath queries or missing options in the cURL setup.
php scraper.php
Step 12: Automate or Schedule
Now that your scraper is functioning properly, consider automating or scheduling it to run at regular intervals. This is particularly useful if you need to scrape data frequently, such as for monitoring price changes or gathering updated content. One common method is to use a cron job on a Unix-based server, which allows you to specify when and how often to run your PHP script.
To set up a cron job, you can open the crontab file with the command:
crontab -e
Then add a line to specify the schedule and the path to your PHP script. For example, to run the scraper every day at 2 AM, you would add:
0 2 * * * /usr/bin/php /path/to/your/scraper.php
Using Headless Browsers for PHP Web Scraping
Thanks to a library called Symfony Panther, you can build a scraper in headless browsers that will be more capable to access web pages that rely on JavaScript rendering (e.g. dynamic content). Panther provides a high-level API for controlling a headless browser, enabling you to interact with web elements, take screenshots, collect data, and navigate complex sites. Here’s how to get started with Symfony Panther for scraping with PHP:
1. Install Symfony Panther: First, ensure you have Composer installed, then add Symfony Panther to your project directory. Run the following command in your terminal:
composer require symfony/panther
Panther will automatically install the necessary dependencies, including a headless browser (like Chrome or Firefox) and the WebDriver.
2. Set Up a basic Panther script: Create a new PHP file (e.g., panther_scraper.php
) and start by including the Composer autoload file. Then, initialize the Panther client to begin scraping.
<?php
require 'vendor/autoload.php';
use Symfony\Component\Panther\Panther;
$client = Panther::createPantherClient();
3. Navigate to a web page: Use the Panther client to navigate to the next page. Panther supports asynchronous requests, allowing you to wait for elements to load.
// Navigate to the target URL
$crawler = $client->request('GET', 'https://example.com');
// Wait for a specific element to load (optional)
$crawler->filter('h1')->count(); // Adjust the selector based on your needs
4. Interact with the page: You can interact with various elements on the page, such as clicking buttons or filling out forms. Panther provides methods to click on elements and input text via the following code:
// Example: Click a button
$crawler->filter('button.submit')->click();
// Example: Fill out a form field
$crawler->filter('input[name="query"]')->sendKeys('search term');
5. Extract data: Once the necessary elements are loaded or interacted with, you can extract data using CSS selectors or XPath queries. Panther's Crawler
object makes it easy to navigate the DOM.
// Extract data from the page
$titles = $crawler->filter('h2.article-title')->each(function ($node) {
return $node->text();
});
// Print the extracted titles
foreach ($titles as $title) {
echo $title . PHP_EOL;
}
6. Close the client: After you've completed the scraping tasks, make sure to close the Panther client to free up resources.
// Close the Panther client
$client->quit();
Web Scraping Challenges and Possible Solutions
Despite the prowess of PHP, there are some web scraping challenges that we need to be mindful of. The most common issues include pagination, CAPTCHAs, and honeypot traps. Thankfully, they can be mitigated via solutions like rotating proxies!
Navigating through Paginated Websites
Symfony Panther can be effectively used to handle pagination in web scraping tasks. When dealing with websites that paginate their content via JavaScript(e.g., articles, products), you can programmatically navigate through the pages and extract data from each one. Here's how to do it:
1. Identify pagination links: First, inspect the target website to determine how pagination is structured. Common patterns include "Next" buttons, numbered page links, or even infinite scroll setups. You’ll need to identify the selectors for these elements.
2. Loop through pages: Use a loop to navigate through the pagination links. You can either click on "Next" links or visit specific numbered pages based on the site's HTML structure. Here’s an example of how to scrape websites with multiple pages using Symfony Panther:
<?php
require 'vendor/autoload.php';
use Symfony\Component\Panther\Panther;
$client = Panther::createPantherClient();
$pageNumber = 1; // Start from the first page
do {
// Navigate to the current page
$crawler = $client->request('GET', 'https://example.com/page/' . $pageNumber);
// Extract data from the current page
$titles = $crawler->filter('h2.article-title')->each(function ($node) {
return $node->text();
});
// Print extracted titles
foreach ($titles as $title) {
echo $title . PHP_EOL;
}
// Check for the "Next" link (assuming it has a specific class)
$nextPage = $crawler->filter('a.next')->count();
$pageNumber++;
} while ($nextPage > 0); // Continue if there is a next page
// Close the Panther client
$client->quit();
3. Handle edge cases: Ensure that you manage edge cases, such as:
- No more pages: Implement checks to break out of the loop when there are no more pages to scrape (as shown in the example).
- Dynamic loading: If the site uses AJAX to load more results (e.g., infinite scrolling), you may need to scroll the web page or simulate clicking a "Load More" button and wait for new content to load.
4. Optimize for performance: If you’re scraping many pages, consider adding delays between requests to avoid overwhelming the server and potentially getting blocked. You can use sleep()
to pause execution briefly:
sleep(1); // Pause for 1 second
Rotating Proxies
Adding proxies with IP rotation to our PHP web scraper is a great way to enhance anonymity and avoid getting blocked by the target website, especially when making multiple requests. Here’s how you can implement rotating proxies in your PHP web scraping script, particularly when using cURL or Symfony Panther.
1. Obtain proxies: Create an Infatica account, sign up for a proxy plan, and get a proxy list.
2. Store proxies: You can store your proxies in an array or a configuration file. A simple approach is to define an array in your PHP script that contains the proxies you want to rotate.
$proxies = [
'http://username:password@proxy1:port',
'http://username:password@proxy2:port',
'http://username:password@proxy3:port',
// Add more proxies as needed
];
3. Randomize proxy selection: Before each request, randomly select a proxy from your list. If you’re using cURL, you can set the CURLOPT_PROXY
option to use the selected proxy.
// Randomly select a proxy
$proxy = $proxies[array_rand($proxies)];
// Initialize cURL session
$ch = curl_init();
// Set cURL options, including the proxy
curl_setopt($ch, CURLOPT_URL, 'https://example.com');
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_PROXY, $proxy);
4. Handle proxy failures: Unfortunately, proxy errors can sometimes occur – and you should implement error handling to switch to a different proxy if the selected one fails. You can do this by checking the response or error codes after executing the cURL session.
$response = curl_exec($ch);
// Check for cURL errors
if (curl_errno($ch)) {
echo 'cURL error: ' . curl_error($ch);
} else {
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
if ($httpCode !== 200) {
echo "HTTP error: " . $httpCode;
// Optionally, remove the failed proxy from the list and retry
}
}
// Close the cURL session
curl_close($ch);
5. Rotating proxies with Symfony Panther: If you’re using Symfony Panther, you can set a rotating proxy in a similar way by configuring the client options. Here’s how you can implement rotating proxies with Panther:
require 'vendor/autoload.php';
use Symfony\Component\Panther\Panther;
// Define your list of proxies
$proxies = [
'http://username:password@proxy1:port',
'http://username:password@proxy2:port',
'http://username:password@proxy3:port',
];
// Randomly select a proxy
$proxy = $proxies[array_rand($proxies)];
// Create a Panther client with the proxy
$client = Panther::createPantherClient([
'proxy' => $proxy,
]);
// Use the client to make requests
$crawler = $client->request('GET', 'https://example.com');
Avoiding Honeypot Traps
Avoiding honeypot traps is crucial for maintaining the effectiveness and integrity of your PHP web scraping pipeline. Honeypots are anti-bot measures set by websites to detect and block bots by presenting fake links or form fields that only bots would interact with.
1. Analyze the target website: Before web scraping with PHP, perform a thorough analysis of the target website to identify patterns that may indicate honeypot traps. Look for:
- Invisible fields: Fields that are hidden from normal users (e.g., with
display: none;
or usingvisibility: hidden;
). - Unusual links: Links that do not seem to lead to real content, such as suspicious or excessively generic URLs.
- Uncommon form fields: Fields with names that are typically used for bots (e.g.,
email
,username
).
2. Implement User-Agent rotation: Many such websites use user-agent detection to identify bots. By rotating user agents, you can make your scraper appear more like a real user. Use a list of common browser user agents and randomly select one for each request.
$userAgents = [
'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3',
'Mozilla/5.0 (Macintosh; Intel Mac OS X 10_12_6) AppleWebKit/605.1.15 (KHTML, like Gecko) Version/14.1.2 Safari/605.1.15',
// Add more user agents as needed
];
$userAgent = $userAgents[array_rand($userAgents)];
curl_setopt($ch, CURLOPT_USERAGENT, $userAgent);
3. Respect robots.txt and crawl delay: Check the website’s robots.txt
file to see which pages you are allowed to scrape. Respect the directives outlined there, and implement a web crawling delay to mimic human behavior, reducing the chance of triggering honeypots.
4. Use randomized delays between requests: Incorporate randomized delays between requests to simulate human browsing behavior. Avoid making requests too quickly or at regular intervals, as this can raise flags.
sleep(rand(1, 5)); // Sleep for a random time between 1 and 5 seconds
5. Avoid unnecessary links and forms: Be selective about the links and forms your scraper interacts with. Avoid clicking on links or submitting forms that seem irrelevant or unrelated to the data you want to scrape. You can maintain a whitelist of URLs or selectors that you know are safe.
6. Monitor for suspicious responses: After submitting forms or clicking links, monitor the responses for suspicious patterns. If the response leads to an HTML page indicating that you’ve been flagged as a bot or requires captcha verification, you should halt further requests.
7. Use headless browsers for complex interactions: For more complex sites that execute JavaScript, consider using headless browsers (like Symfony Panther or Puppeteer). They can simulate real user interactions more effectively and may help avoid detection.
8. Test your scraper regularly: Regularly test and update your scraper to adapt to any changes in the target website’s structure. By keeping your scraper flexible and responsive, you can reduce the risk of falling into honeypot traps.
9. Analyze scraping patterns: Keep logs of your scraping activities and analyze patterns to identify any triggers that might lead to honeypots. Adjust your scraping strategy based on these insights.
10. Implement error handling: Implement robust error handling to manage responses indicating that your scraper has been blocked. You may choose to back off or add proxy rotation if you detect repeated failures.