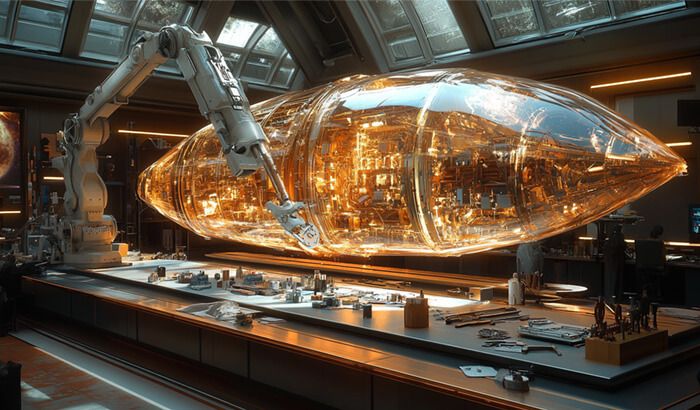
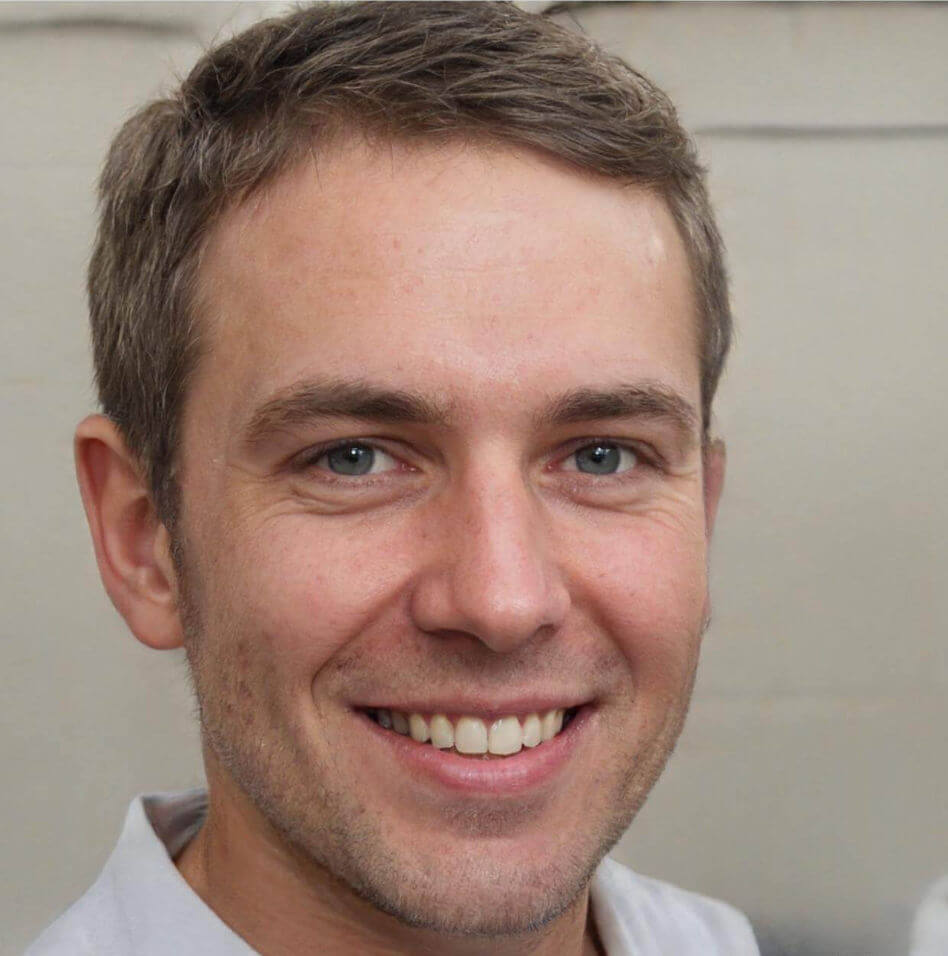
Job posting platforms contain a lot of valuable data – and if you scrape it efficiently, you can derive valuable insights into job market trends, company hiring strategies, and evolving skill demands. Whether you use simple tools like Excel or powerful databases and visualization platforms, organizing and interpreting your data will unlock its full potential – let’s learn how to scrape this data in our comprehensive guide!
Why scrape job postings?
Having access to up-to-date information is crucial for both job seekers and employers. Web scraping offers a powerful way to gather vast amounts of job data quickly and efficiently. But why would someone want to scrape job postings in the first place? Let’s break it down.
Job seekers: gaining a competitive edge
For job seekers, web scraping can be a game changer. Instead of manually checking multiple job boards every day, scraping allows users to gather listings from several sources at once, saving time and effort. By automating this process, job seekers can:
- Stay informed on new postings: Fresh listings can be scraped and compiled as soon as they appear online, ensuring that candidates don’t miss out on any opportunities.
- Analyze job trends: Scraping job descriptions can reveal insights into what skills and qualifications are currently in demand. This helps job seekers tailor their resumes or focus on acquiring specific expertise that is sought after.
- Target specific companies: Scraping allows users to monitor specific employers for new job postings, making it easier to catch openings at preferred companies.
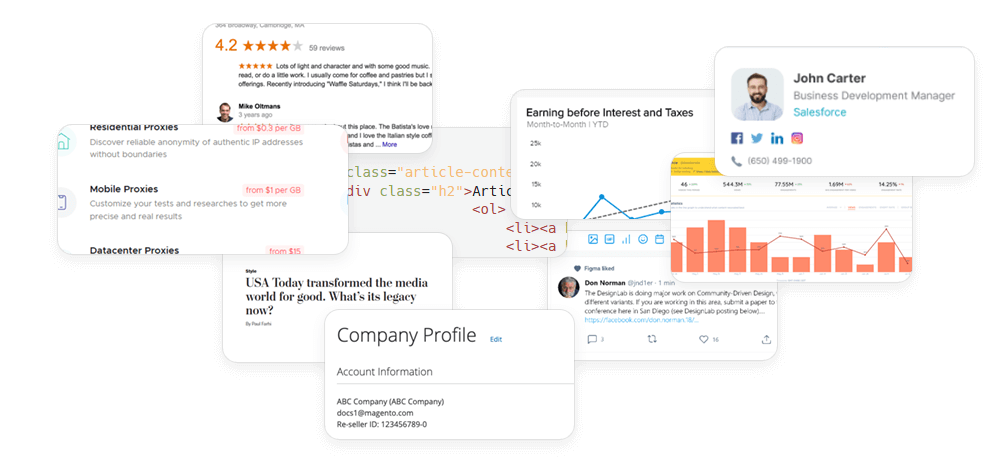
Businesses: market insights and competitive analysis
For businesses, the benefits of scraping job postings extend beyond just recruitment. Companies can use scraped data to stay competitive in their industry. Here’s how:
- Recruitment intelligence: By scraping job postings from competitors, businesses can track the roles they’re hiring for, the qualifications they’re seeking, and the salary ranges offered. This can help HR departments craft more competitive offers and identify gaps in their own workforce.
- Market trends: Scraping thousands of job postings can reveal larger patterns in hiring practices across industries or regions. This data can help businesses make strategic decisions about talent acquisition or expansion into new markets.
- Identifying skills gaps: By analyzing what skills are frequently listed in job postings, businesses can identify areas where their workforce might need upskilling or additional training.
Data for researchers and analysts
Beyond job seekers and businesses, scraped job data can also be a valuable resource for researchers, economists, and market analysts. For instance:
- Economic forecasting: Analyzing job postings over time can provide insights into which industries are growing or shrinking. It can also help identify regional job market trends or skill shortages.
- Labor market research: Researchers often rely on scraped job data to conduct studies on employment trends, workforce skills, and salary distribution across different sectors.
- Policy analysis: Governments and policy think tanks can use scraped data to evaluate the effectiveness of employment policies and programs by tracking hiring trends and job availability.
Choosing the right tools
When it comes to scraping job postings, selecting the right tool can make all the difference in efficiency, ease of use, and scalability. Below is a comparison of some of the most popular web scraping tools, each with its strengths and weaknesses depending on the user’s technical expertise and the complexity of the scraping task.
Tool | Language | Ease of Use | Key Features | Best Suited For | Limitations |
---|---|---|---|---|---|
Beautiful Soup | Python | Easy | Simple to use for beginners. Parses HTML/XML efficiently. Works well with static pages. | Beginners or small projects | Can be slow for large-scale scraping. Lacks advanced features. |
Scrapy | Python | Moderate | Full-fledged scraping framework. Asynchronous scraping for faster data collection. Built-in data pipelines. | Large-scale, complex scraping projects | Steeper learning curve. Requires more setup. |
Selenium | Multiple (Python, Java, etc.) | Moderate | Handles JavaScript-heavy/dynamic content. Simulates real user behavior by controlling a web browser. | Scraping dynamic websites with JS content | Slower performance. Requires more resources (memory/CPU). |
MechanicalSoup | Python | Easy | . Simulates form submissions and logins. Ideal for small tasks requiring automation. | Automating simple, form-based scraping | Less efficient for large-scale scraping. Limited compared to Scrapy. |
Key considerations when choosing a tool:
- Technical expertise: If you have coding experience, tools like Scrapy or Beautiful Soup offer more flexibility and control. Non-technical users may prefer GUI-based tools.
- Project size: For small-scale, one-time scraping, Beautiful Soup might be enough. For large, ongoing projects, tools like Scrapy are more suitable due to their scalability.
- Handling dynamic content: For websites that heavily use JavaScript (such as job sites with dynamically loaded postings), Selenium or a cloud-based scraper is ideal.
- Speed and efficiency: If scraping speed is a priority, tools with asynchronous capabilities like Scrapy outperform browser-based solutions like Selenium.
Guide: Scraping a job posting page
Before we start, ensure you have the following Python libraries installed:
pip install requests beautifulsoup4 pandas
1. Identify the target webpage. For this example, let's assume we are scraping a generic job listing page, such as https://example.com/jobs
.
2. Inspect the website structure. Using the browser's Inspect tool (right-click on the page and select "Inspect"), find the HTML tags containing the job title, company name, and location. For instance, you might find that:
- Job title is in an
<h2>
tag with the classjob-title
- Company name is in a
<span>
tag with the classcompany-name
- Location is in a
<span>
tag with the classjob-location
3. Fetch the webpage content. Here’s how to use Python's requests
library to fetch the HTML content of the job postings page:
import requests
# URL of the job listings page
url = 'https://example.com/jobs'
# Send a GET request to fetch the page content
response = requests.get(url)
# Check if the request was successful
if response.status_code == 200:
page_content = response.text
else:
print(f"Failed to retrieve the webpage. Status code: {response.status_code}")
4. Parse the HTML with Beautiful Soup. Now, let’s use Beautiful Soup to parse the HTML and extract the job data:
from bs4 import BeautifulSoup
# Parse the HTML content using BeautifulSoup
soup = BeautifulSoup(page_content, 'html.parser')
# Find all job postings (assuming they are in 'div' tags with the class 'job-listing')
job_listings = soup.find_all('div', class_='job-listing')
# Extract job title, company, and location for each job posting
jobs = []
for listing in job_listings:
job = {
'title': listing.find('h2', class_='job-title').text.strip(),
'company': listing.find('span', class_='company-name').text.strip(),
'location': listing.find('span', class_='job-location').text.strip(),
}
jobs.append(job)
# Print the job postings
for job in jobs:
print(job)
5. Store the data in a CSV file. Once the data is scraped, it can be stored in a CSV file for further analysis. Here’s how:
import pandas as pd
# Convert the list of job postings into a DataFrame
jobs_df = pd.DataFrame(jobs)
# Save the DataFrame to a CSV file
jobs_df.to_csv('job_postings.csv', index=False)
print("Job data saved to 'job_postings.csv'")
6. Example output. After running this script, you will get a CSV file with the following structure:
title | company | location |
---|---|---|
Software Engineer | Tech Corp | New York, NY |
Data Analyst | Data Solutions | San Francisco, CA |
Project Manager | Innovate Ltd | Chicago, IL |
Dynamic content: If the job listings are loaded dynamically with JavaScript, you'll need to use Selenium to scrape them (as explained previously). Here’s a quick snippet for Selenium:
from selenium import webdriver
from bs4 import BeautifulSoup
# Set up Selenium and open the webpage
driver = webdriver.Chrome()
driver.get('https://example.com/jobs')
# Get the page source after dynamic content is loaded
page_source = driver.page_source
# Parse the page with BeautifulSoup
soup = BeautifulSoup(page_source, 'html.parser')
# Continue as usual with finding job listings and extracting data
Handle rate limiting: To avoid overloading the server, add a delay between requests using time.sleep()
. For instance, a delay of 2 seconds:
import time
time.sleep(2) # Sleep for 2 seconds between requests
Common challenges in web scraping
Web scraping job postings can be highly effective, but it’s not without challenges. From complex site structures to anti-scraping mechanisms, websites often make it difficult to extract data consistently and efficiently. Let’s explore some of the most common obstacles you may encounter when scraping job sites, and discuss solutions to address them.
1. Dynamic content
Many modern websites use JavaScript to dynamically load content, which means the HTML you see in your browser isn’t fully available when you send an HTTP request to the site. Instead of returning static HTML, the server delivers a page skeleton, and the content (such as job listings) is loaded afterward by JavaScript code.
Example: On a job site, you may notice that as you scroll down, more job postings load. This is called infinite scrolling, and it’s powered by JavaScript.
Challenge: When you use traditional libraries like requests
to fetch a page, the dynamically loaded content may not appear in the HTML response.
Solution: Tools like Selenium and Playwright can handle dynamic content by simulating a real browser. These tools allow you to interact with pages as a user would (e.g., clicking buttons, scrolling down) and capture the rendered HTML after the content has fully loaded.
from selenium import webdriver
driver = webdriver.Chrome()
driver.get("https://example.com/jobs")
page_source = driver.page_source # HTML after JavaScript execution
2. AJAX
AJAX (Asynchronous JavaScript and XML) is a technique that allows websites to fetch data in the background without reloading the page. Many job boards use AJAX to update listings dynamically when users search or apply filters.
Example: A job search page may allow you to refine your search with filters (e.g., location, salary range), and these filters trigger AJAX requests to fetch filtered results.
Challenge: If you're scraping a page with AJAX, the data might not be present in the initial HTML response. Instead, it's loaded asynchronously by AJAX calls.
Solution: By inspecting the network requests in your browser’s Developer Tools, you can often find the specific API that the AJAX call is using. Once identified, you can scrape data directly from that API instead of the main HTML page. This approach is faster and more efficient.
import requests
# Example of directly hitting an API endpoint used by AJAX
api_url = "https://example.com/api/jobs"
response = requests.get(api_url)
data = response.json() # Get job listings as JSON
3. robots.txt
The robots.txt
file provides guidance on which pages can or cannot be crawled by bots. While not legally binding, it’s a widely respected standard in the web community. For example, some job sites may disallow scraping of job posting pages.
Example: Checking https://example.com/robots.txt
may reveal that the /jobs
path is disallowed for bots.
User-agent: *
Disallow: /jobs
Challenge: While robots.txt
won’t actively block your scraper, ignoring it can lead to legal or ethical issues.
Solution: Always check and respect the robots.txt
file to ensure your scraping activities comply with the site’s rules.
4. IP blocking
Websites often monitor the rate and frequency of incoming requests. If they detect abnormal traffic from a single IP address (such as a scraper making hundreds of requests in a short time), they may block that IP temporarily or permanently.
Challenge: Once your IP is blocked, you won’t be able to access the site’s content.
Solution: IP rotation is a common strategy to bypass IP blocking. By using a pool of proxy IPs, you can distribute your requests across different addresses to avoid detection. Here's how to use the requests
library with a proxy:
proxies = {
"http": "http://your_proxy_ip:port",
"https": "http://your_proxy_ip:port"
}
response = requests.get("https://example.com/jobs", proxies=proxies)
5. CAPTCHAs
CAPTCHAs are designed to verify whether the user accessing a website is a human or a bot. They can be triggered by unusual traffic patterns, such as scraping.
Challenge: CAPTCHAs will block your scraper from accessing further content until solved, effectively halting your scraping operation.
Solution: While CAPTCHAs are difficult to bypass, you can use third-party CAPTCHA-solving services, such as 2Captcha or Anti-Captcha, to automate the process.
# Example integration with 2Captcha (for complex cases)
import requests
api_key = "your_2captcha_api_key"
captcha_solution = requests.post("https://2captcha.com/solve", data={"key": api_key, "url": "https://example.com"}).json()
Alternatively, if available, you can use an API provided by the website to access the same data without triggering CAPTCHAs. Example: Some job boards provide APIs where you can query job postings directly. Here’s a basic API call example:
api_url = "https://example.com/api/jobs"
response = requests.get(api_url, params={"location": "New York", "job_type": "Software Engineer"})
job_data = response.json() # Parse the JSON response
Analyzing and storing the data
Once you've successfully scraped job postings, the next step is to turn that raw data into meaningful insights. Let’s explore how to analyze the data to uncover trends in the job market, as well as the tools and methods you can use to store and visualize the data effectively.
Tools for storing the data
After scraping job data, the next step is to store it in a format that allows for easy access and analysis. Here are some of the common tools for storing scraped data:
1. CSV (Comma-Separated Values):
- Use case: Ideal for small to medium-sized datasets, easy to manipulate with spreadsheet software (e.g., Excel, Google Sheets).
- Storage: CSV files are lightweight and easy to share, but they don’t support advanced querying.
- Example: After scraping job postings, you can save them into a CSV file for easy access:
import csv
with open('job_postings.csv', 'w', newline='', encoding='utf-8') as csvfile:
fieldnames = ['title', 'company', 'location', 'salary', 'date_posted']
writer = csv.DictWriter(csvfile, fieldnames=fieldnames)
writer.writeheader()
for job in jobs:
writer.writerow(job)
2. Excel:
- Use case: Excel is excellent for small to mid-sized data analysis. It’s particularly useful for users who are comfortable with Excel’s functions, filters, and pivot tables.
- Storage: Data is stored in workbooks and can be analyzed using built-in tools, such as graphs and charts.
- Example: You can use Excel to create pivot tables to summarize job postings by location or role, or generate charts to visualize trends.
3. SQL databases:
- Use case: For larger datasets or when complex querying and relationships between datasets are needed, SQL databases (e.g., MySQL, PostgreSQL, SQLite) are ideal.
- Storage: SQL databases are structured and support powerful querying, making them suitable for more complex analyses (e.g., filtering, aggregating, joining tables).
- Example: After scraping, you can store job postings in a SQL database for further querying:
import sqlite3
# Connect to (or create) a database
conn = sqlite3.connect('jobs.db')
c = conn.cursor()
# Create a table for job postings
c.execute('''CREATE TABLE IF NOT EXISTS job_postings
(title TEXT, company TEXT, location TEXT, salary TEXT, date_posted TEXT)''')
# Insert job data
for job in jobs:
c.execute("INSERT INTO job_postings (title, company, location, salary, date_posted) VALUES (?, ?, ?, ?, ?)",
(job['title'], job['company'], job['location'], job.get('salary', ''), job.get('date_posted', '')))
conn.commit()
conn.close()
4. NoSQL databases:
- Use case: For unstructured or semi-structured data (e.g., job postings with varying fields), NoSQL databases like MongoDB are a good choice.
- Storage: NoSQL databases are flexible and allow you to store JSON-like documents without a predefined schema.
- Example: Store job postings as documents in MongoDB:
from pymongo import MongoClient
# Connect to MongoDB
client = MongoClient('localhost', 27017)
db = client['job_database']
job_collection = db['job_postings']
# Insert job postings
job_collection.insert_many(jobs)
Tools for visualizing the data
Visualizing data helps uncover patterns and insights that might not be immediately obvious from the raw data. Here are some tools to help you visualize job market trends and other insights:
1. Matplotlib and Seaborn (Python):
- Use case: Great for creating custom data visualizations (e.g., bar charts, line graphs, heatmaps).
- Example: Visualize the distribution of job postings by location:
import matplotlib.pyplot as plt
import seaborn as sns
# Create a bar plot of job postings by location
job_locations = [job['location'] for job in jobs]
sns.countplot(y=job_locations)
plt.title('Job Postings by Location')
plt.show()
2. Tableau:
- Use case: A powerful tool for creating interactive dashboards and visualizations, Tableau makes it easy to share insights with others.
- Example: After importing your job data into Tableau, you can create dynamic dashboards that filter jobs by title, location, or company.
3. Power BI:
- Use case: Similar to Tableau, Power BI allows you to create interactive visualizations and reports. It integrates well with Microsoft tools like Excel and SQL Server.
- Example: Import your job data and create interactive reports showing trends in job demand across industries or regions.
4. Google Data Studio:
- Use case: For a free, web-based tool, Google Data Studio offers robust reporting and visualization capabilities. It can pull data from Google Sheets, CSV files, and other sources.
- Example: Use Google Sheets to store your job postings, then connect the data to Google Data Studio to create live, shareable dashboards.