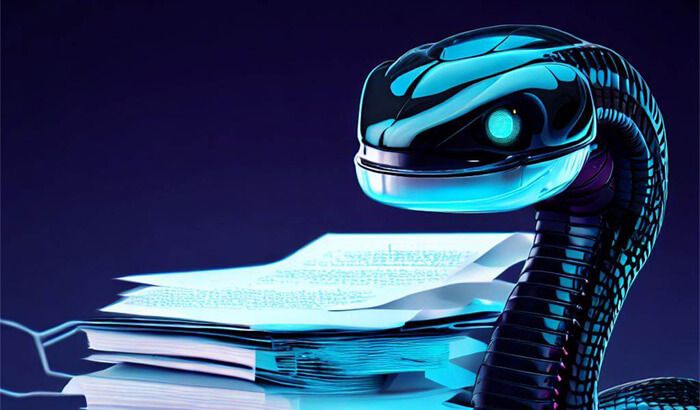
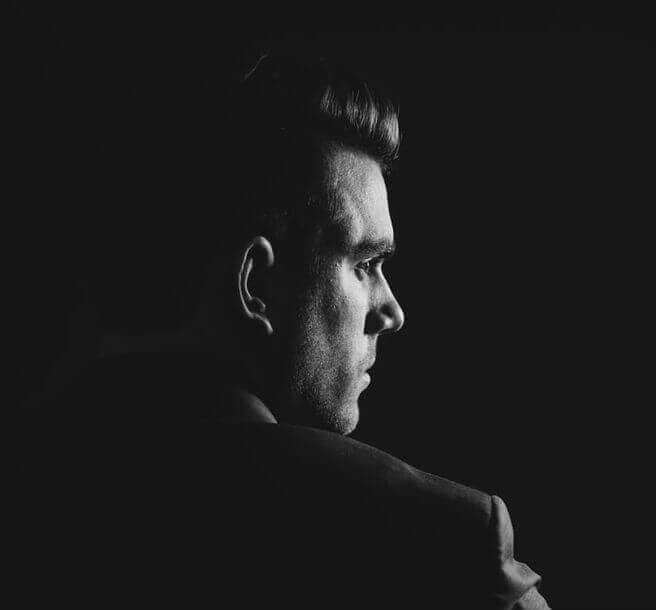
lxml is a powerful and easy-to-use library for processing XML and HTML in Python which offers a fast and feature-rich interface to the libxml2 and libxslt libraries – these are widely used for parsing, validating, and transforming XML documents. In this Python lxml tutorial, you will learn how to use lxml to create, parse, and query XML and HTML documents with various examples. You will also learn how to use lxml for web scraping, data extraction, and data analysis. By the end of this lxml Python tutorial, you will be able to use lxml for your own data processing and data parsing projects.
What is lxml?
The lxml library allows for easy handling of XML and HTML files, providing a fast and feature-rich interface to these libraries using the ElementTree API, which is a simple and standard way of working with XML trees in Python. This is a Pythonic binding for the C libraries libxml2 and libxslt, which are widely used for parsing, validating, and transforming XML documents.
The lxml Python library extends the ElementTree API significantly to offer support for various XML features and standards, such as XPath, RelaxNG, XML Schema, XSLT, C14N, and much more. lxml also supports HTML parsing and web scraping, as well as custom XML element classes and Python extension functions for XPath and XSLT.
lxml has numerous advantages: It's easy to install and use, and it is compatible with all CPython versions from 2.7 to 3.9. It's also well documented and has a large and active user community. lxml is the most powerful and comprehensive library for processing XML and HTML in the Python language.
Install lxml
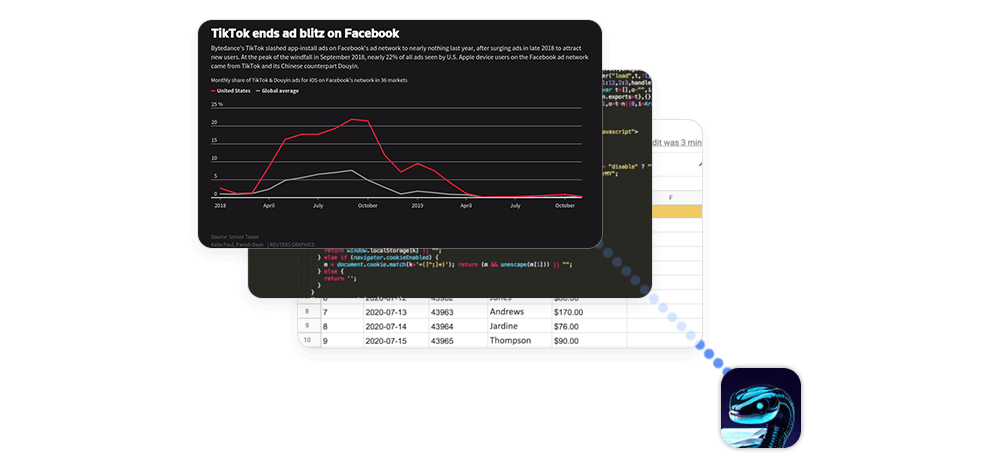
We have a few options of installing the Python lxml library. For Windows users, the most popular method is pip
, Python’s package manager. You can run the second command to install a specific version:
pip install lxml
pip install lxml==4.9.2
Linux (first command below) and macOS (second command below) users can also utilize their system’s native package managers to install lxml:
sudo apt-get install python3-lxml
sudo port install py27-lxml
Create XML and HTML documents
The lxml etree
module offers the core functionality of the library – let’s import it to start creating and working with XML/HTML documents:
from lxml import etree as et
The code snippet below uses etree
in lxml to generate the basic structure of an HTML file. The Element
function creates an element with a given name and any number of attributes. It takes one parameter, the element name. The SubElement
function creates a child element under a root node. It takes two parameters: the root node and the child element name. You can add attributes to any element by passing extra parameters in the format of AttributeName=‘AttributeValue’
.
root = et.Element('html', version="5.0")
# Pass the parent node, name of the child node,
# and any number of optional attributes
et.SubElement(root, 'head')
et.SubElement(root, 'title', bgcolor="black", fontsize='20')
et.SubElement(root, 'body', fontsize="14")
Use lxml to parse XML and HTML documents
We can also use the lxml parser to process existing XML and HTML files, extracting their attributes and data. Let’s use the HTML page we created earlier and apply this code snippet:
for e in root:
print(e.tag)
Upon running this code, we’ll see the HTML tags of all child elements from the root node: head
, title
, and body
.
Add element attributes
We can use lxml to assign attributes to previously created elements – and retrieve them from any element. This Python lxml example code will add a sample attribute titled SampleAttribute
with the sample value of AttributeValue
to the root element:
root.set('SampleAttribute', 'AttributeValue')
# Print root again to test if the attribute has been added
print(et.tostring(root, pretty_print=True).decode("utf-8"))
Here’s the output. The attribute has been added successfully:
<html version="5.0" SampleAttribute="AttributeValue">
<head/>
<title bgcolor="red" fontsize="22"/>
<body fontsize="15"/>
</html>
If we want to retrieve another attribute’s value, we’ll need the get()
method. Upon running this code, we’ll have this list of attributes: AttributeValue
, None
, black
:
print(root.get('SampleAttribute'))
print(root[1].get('alpha')) # root[1] accesses the `title` element
print(root[1].get('bgcolor'))
Get text data from elements
We’ve been using the lxml.etree
module to retrieve metadata like HTML tags – but we can also collect the text data (e.g. labels, descriptions, and titles) stored within HTML:
root = et.Element('html', version="5.0")
et.SubElement(root, 'head')
et.SubElement(root, 'title', bgcolor="black", fontsize="20")
et.SubElement(root, 'body', fontsize="14")
# Add text to the Elements and SubElements
root.text = "HTML file description"
root[0].text = "Head of the HTML file"
root[1].text = "Title of the HTML file"
root[2].text = "Body of the HTML file"
print(et.tostring(root, pretty_print=True).decode("utf-8"))
Here's the output:
<html version="5.0">HTML file description<head>Head of the HTML file</head><title bgcolor="black" fontsize="20">Title of the HTML file</title><body fontsize="14">Body of the HTML file</body></html>
Check the element’s children
lxml can help us with an important task: checking if the given element has any children. This will determine how we can access and manipulate the data in the element: For example, if an element has children, you can use methods like findall
, xpath
, or list
to get the child elements and their text or attributes. If an element has no children, you can only access its own text or attributes.
This code snippet will print True
because there are child nodes tied to the root node:
if len(root) > 0:
print("True")
else:
print("False")
Conversely, this code snippet will print False
because the child nodes don’t have children of their own:
for i in range(len(root)):
if (len(root[i]) > 0):
print("True")
else:
print("False")
Check the element’s parent
Similarly, if an element has a parent, you can use methods like getparent
or find
to get the parent element and its properties. If an element has no parent, it means it is the root of the document and you cannot go up any further in the tree. In this code snippet, for example, each line in this code snippet looks for the node’s parent element:
print(root.getparent())
print(root[0].getparent())
print(root[1].getparent())
Here’s the output:
None
<Element html at 0x1103c9688>
<Element html at 0x1103c9688>
The first element is the root node, so we get None. In line two and three, we get referenced to their root elements.
Conclusion
In this lxml tutorial, you’ve learned how to use lxml for web scraping in Python. lxml is a fast and powerful library that allows us to parse HTML and XML documents and extract their data. Web scraping with lxml is a useful skill that can help us create our own datasets, analyze web data, or automate tasks – stay tuned for more data collection-related guides!
Frequently Asked Questions
HTMLParser
class, the html5parser
module, or the lxml.html
module to help lxml with HTML processing. They can handle broken tags, missing attributes, and other common errors.
xpath()
method of the Element
or ElementTree
classes to evaluate XPath expressions on XML or HTML documents. You can also use the XPath
class to compile and reuse XPath expressions.
nsmap
argument of the Element
or SubElement
classes to create custom XML namespaces with lxml. You can also use the QName
class to create qualified names with namespaces.
tostring()
or tounicode()
functions to serialize an XML or HTML tree to a string. You can also use the write()
method of the ElementTree
class to serialize an XML or HTML tree to a file.
XMLSchema
or DTD
classes to validate XML documents against a schema or a DTD. You can also use the RelaxNG
or Schematron
classes to validate XML documents against other schema languages.
XSLT
class to apply XSLT transformations to XML documents with lxml. You can also use the xslt()
method of the ElementTree
class to apply XSLT transformations in one step.
Comment
or ProcessingInstruction
classes to create and access XML comments and processing instructions with lxml. You can also use the itercomment()
or iterpi()
methods of the ElementTree
class to iterate over them.
lxml.etree.libxml2_version
and lxml.etree.libxslt_version
attributes to get the version numbers of the underlying libxml2 and libxslt libraries. You can also use the lxml.etree._Element
and lxml.etree._XSLTResultTree
classes to access their low-level C structures.
parse()
, tostring()
, tounicode()
, or write()
functions to specify the encoding of XML or HTML documents. You can also use the recover
argument of the XMLParser or HTMLParser
classes to handle encoding errors gracefully.